Making an API call
PHP Code
This sample code shows how to make a GET request to the Attom property API to receive property details. It is important to note that you should input your API key in the headers defined. See the example below:
"accept: application/json",
"apikey: ENTER YOUR API KEY HERE",
CURL Example
You can adjust the address by adjusting the address1 and address2 field in the URL here:
CURLOPT_URL => "https://api.gateway.attomdata.com/propertyapi/v1.0.0/property/detail?address1=4529%20Winona%20Court&address2=Denver%2C%20CO",
See our documentation page for other endpoints and filtering options here - https://api.developer.attomdata.com/docs
Check out this sample code in Github at
https://github.com/Onboard-Informatics/Sample_Code/blob/master/Property_Detail.php
import http.client
conn = http.client.HTTPSConnection("api.gateway.attomdata.com")
headers = {
'accept': "application/json",
'apikey': "",
}
conn.request("GET", "/propertyapi/v1.0.0/property/
detail?address1=4529%20Winona%20Court&address2=
Denver%2C%20CO", headers=headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
<?
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.gateway.attomdata.com/propertyapi/v1.0.0/
property/detail?address1=4529%20Winona%20Court&address2=
Denver%2C%20CO",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"apikey: ",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
?>
require 'uri'
require 'net/http'
url = URI("https://api.gateway.attomdata.com/propertyapi/
v1.0.0/property/detail?address1=4529%20Winona%20Court&
address2=Denver%2C%20CO")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
request["accept"] = 'application/json'
request["apikey"] = ''
response = http.request(request)
puts response.read_body
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://api.gateway.attomdata.com/propertyapi/v1.0.0/
property/detail?address1=4529%20Winona%20Court&address2=
Denver%2C%20CO")
.get()
.addHeader("accept", "application/json")
.addHeader("apikey", "")
.build();
Response response = client.newCall(request).execute();
Schools on Google Maps
After following this guide and integrating the API with Google Maps in your product, you will be able to display a pin overlay the map image to show where a school is located. The marker in this guide is the standard marker image from Google
1. The very first step would be setting up your product to hit the API through cURL in order to pull back location information about the schools within overlapping attendance zones of the address entered.
a. Here is an example API URL : Click here
2. This API call returns an array containing property information along with specific details about schools in the applicable attendance zones around the property.
3. The next step is to initialize the Google Map per the same requested address. You can find details about that process here google map api.
4. Once you have completed the map initialization, then you need to set the marker pins per the latitude and longitude for the school that you want to display.
a. Here is the example of set marker pins : marker sample
5. Once you’re done with the marker pin setup, then this code should help you display the Attom school data on your Google map display for your product.
Code snippet not available in Ruby on Python (Coming Soon)
Code snippet not available in Php (Coming Soon)
Code snippet not available in Ruby on rails (Coming Soon)
<? curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.gateway.attomdata.com
/propertyapi/v1.0.0/property/detailwithschools
?address1=4529%20Winona%20Court&address2=Denver%2C%20CO",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"apikey: ",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
?>
<script>
var gmarkers = [];
function initMap() {
var locations = [<?php
if(!empty($final_array)) {
$ps = 1;
foreach($final_array as $publicSchool){
if ($publicSchool['InstitutionName'] !=''){ ?>
['<?php echo $publicSchool['InstitutionName'];
?>',
'<?php echo $publicSchool['geocodinglatitude'];
?>',
'<?php echo $publicSchool['geocodinglongitude'];
?>',
'<?php echo $ps; ?>','
<?php echo $publicSchool
['InstitutionName']; ?>
<?php echo $publicSchool['school_address']
['locationaddress']." ".
$publicSchool['school_address']['locationcity']
." ".
$publicSchool['school_address']['stateabbrev']
.", ".
$publicSchool['school_address']['ZIP']; ?>
<?php echo $publicSchool
['distance']; ?>
Miles
'],
<?php $ps++; ?>
<?php } ?>
<?php } ?>
<?php } ?>
];
var map = new google.maps.Map(document.getElementById
('map'),
{
zoom: 12,
center: new google.maps.LatLng('<?php echo
$sourceLocationLatitude; ?>',
'<?php echo $sourceLocationLongitude; ?>'),
mapTypeId: google.maps.MapTypeId.ROADMAP
});
var infowindow = new google.maps.InfoWindow();
var marker, i;
marker = new google.maps.Marker({
position: new google.maps.LatLng('<?php echo
$sourceLocationLatitude; ?>',
'<?php echo $sourceLocationLongitude; ?>'),
map: map,
animation: google.maps.Animation.DROP,
icon: 'images/4.png'
});
gmarkers.push(marker);
for (i = 0; i < locations.length; i++) {
marker = new google.maps.Marker({
position: new google.maps.LatLng(locations[i][1],
locations[i][2]),
map: map,
animation: google.maps.Animation.DROP,
icon: 'images/1.png'
});
google.maps.event.addListener(marker, 'click',
(function(marker, i) {
return function() {
infowindow.setContent(locations[i][4]);
infowindow.open(map, marker);
for (var sm = 0; sm < gmarkers.length;
sm++) {
if(sm!=0){
gmarkers[sm].
setIcon("images/1.png");
}
}
marker.setIcon("images/2.png");
}
})(marker, i));
gmarkers.push(marker);
}
}
function openInfoModal(i) {
google.maps.event.trigger(gmarkers[i], "click");
}
$(function(){
initMap();
});
</script>
Using Google Auto-complete to search the Attom API
If you aren’t already aware of the functionality available in the Google location autocomplete web service, you can view all of the documentation here.
One very popular implementation for our partners is to make this service the first step in the process of collecting an address from their customers to ensure that it is valid and in good shape to pass to the API.
In this guide, we will walk you through the necessary steps to take an address from the output of the Google location autocomplete web service and pass it to the Attom API to collect property details.
Getting Started
The first step in the process is to ensure that you have the Google web service set up and in place on your application. You can find all of the steps for implementing the web service here - Google Places Example.
What comes next after getting the google location address response
Once you receive the suggested address, you need to break the address into two pieces to pass to the Attom API. The Attom API accepts two parameters called “address1” and “address2” to search for a property. This can be done through separation via comma(,) values and the code below uses “4529 Winona Court, Denver CO United States 80212” address as example.
Now you’re all set to start pulling data from the Attom API. All of the different available API calls, response bodies, filtering options and interactive docs can be found in the API Docs page. This will help you construct the right response to feed your product!
Code snippet not available in Ruby on Python (Coming Soon)
Code snippet not available in Ruby on Ruby on rails (Coming Soon)
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<meta charset="utf-8">
<title>Using Google Auto-complete to search the Attom API</title>
</head>
<body>
<form method="post">
<input id="pac-input" class="controls" name="google_address" type="text" placeholder="Search Box">
<input type="submit" name="submit" value="Submit"/>
</form>
<script>
function initAutocomplete() {
// Create the search box and link it to the UI element.
var input = document.getElementById('pac-input');
var searchBox = new google.maps.places.SearchBox(input);
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=GOOGLE_API_KEY&libraries=places&callback=initAutocomplete"
async defer></script>
</body>
</html>
<?php
if(isset($_REQUEST['submit']) AND ($_REQUEST['submit'] =="Submit")){
$suggestive_address = (isset($_REQUEST['google_address']) && $_REQUEST['google_address'] !="")?$_REQUEST['google_address']:'4529 Winona Court, Denver CO United States 80212';
$explodedAddressArr = explode(",", $suggestive_address);
//Example value : 4529 Winona Court
$address1 = urlencode($explodedAddressArr[0]);
//Example value : Denver CO United States 80212
$address2 = urlencode($explodedAddressArr[0]);
/*Once the address is separated, you can pass these parameters into the Attom API using cURL hit.*/
$url = "https://api.gateway.attomdata.com/propertyapi/v1.0.0/property/detailwithschools?address1=$address1&address2=$address2";
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"apikey: USE_YOUR_ATTOM_API_KEY_HERE"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if($err){
echo '{"status": { "code": 999, "msg": "cURL Error #:" . $err."}}';
}else{
echo $response;
}
}
?>
School Data Sample
PHP Code
If you’re looking for a clean and simple design to show Attom's school data on your website, check out the sample that we have created to save your time on implementation. Here are the basic steps you can use to get started:
1. Take a look at the demo page here and test drive it by entering a few addresses - view demo
2. If you like what you see, get signed up for a free trial API key by signing up here. This will give you 90 days to implement the API and make sure that you have time to complete testing.
3. Once you have a trial API key, click here to get the Github Gists for this page. The gists available include the following:
a. Source Code (e.g. PHP file for pulling the right data from the API)
b. HTML Code (for the visualization of the data)
c. Javascript Code (for the page functionality)
4. Use the gists to implement the demo page as shown or customize the data, colors, and functionality to fit your needs.
5. Once you are done testing, contact us to get started with a production API key! Here are a few highlights about the page:
- All of the schools listed at the top of the screen are located within the attendance zone for the property being searched.
- You can select the schools on the top slider or on the map to show details about the school.
- Private schools within a 10-mile radius can also be found by scrolling through the schools on the slider. (private schools are shown with the $ on the public vs. private indicator)
Code snippet not available in Ruby on Python (Coming Soon)
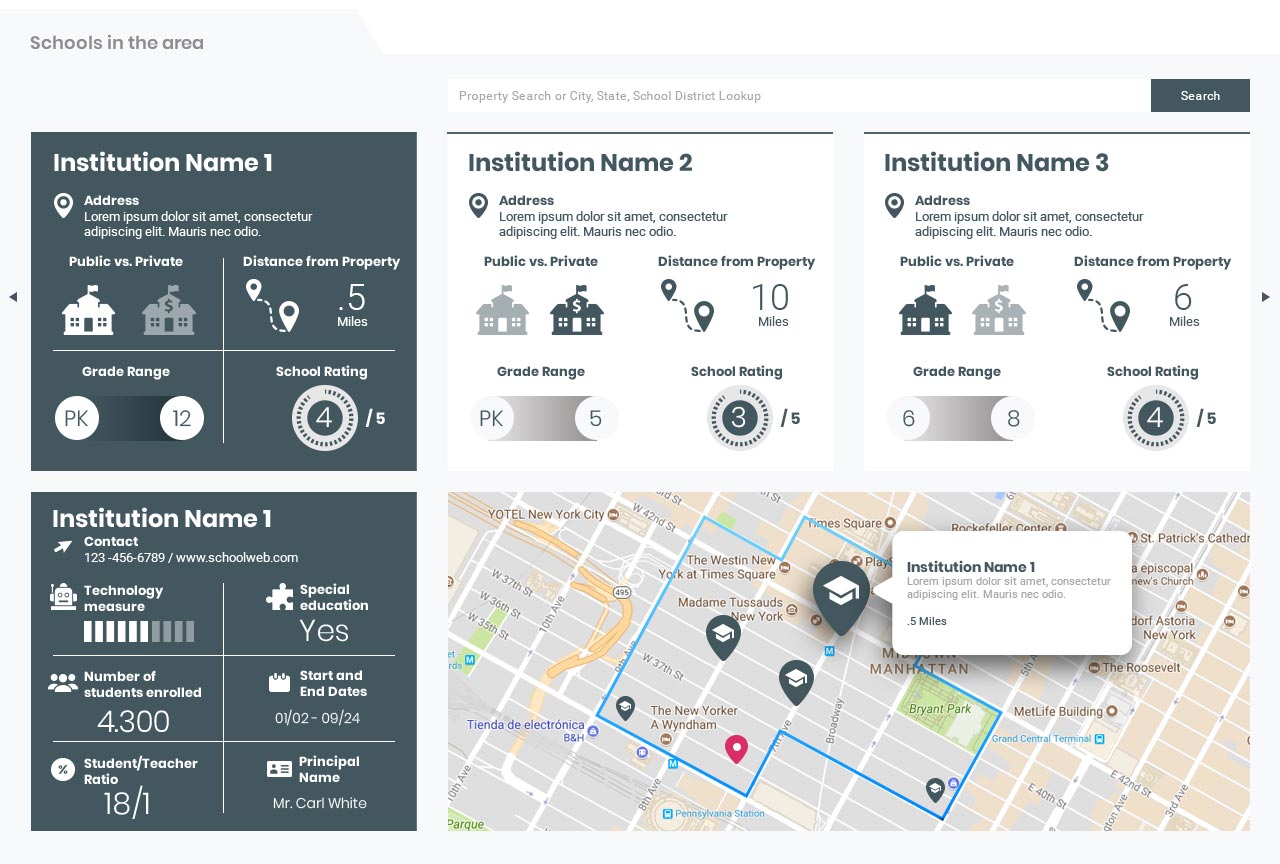
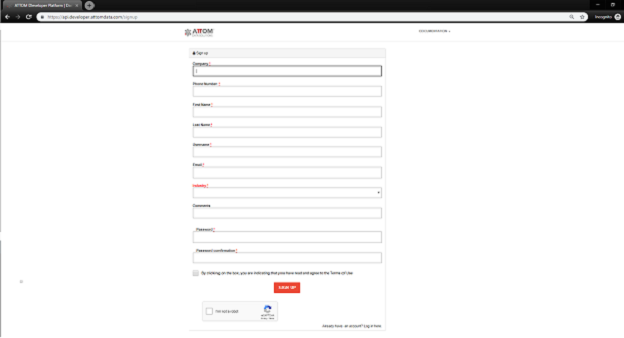
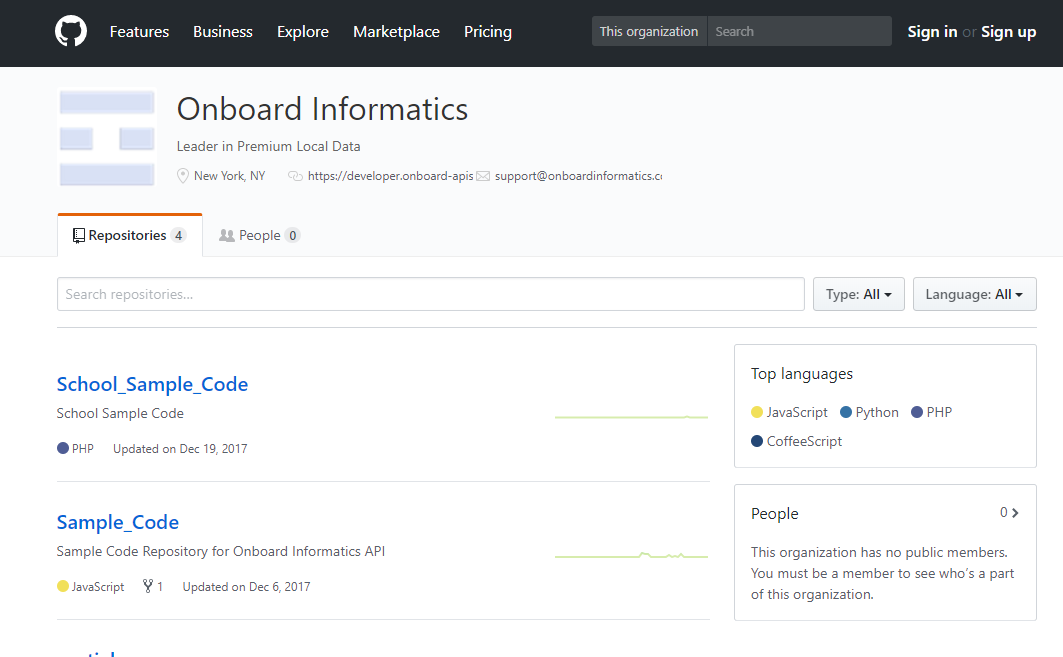
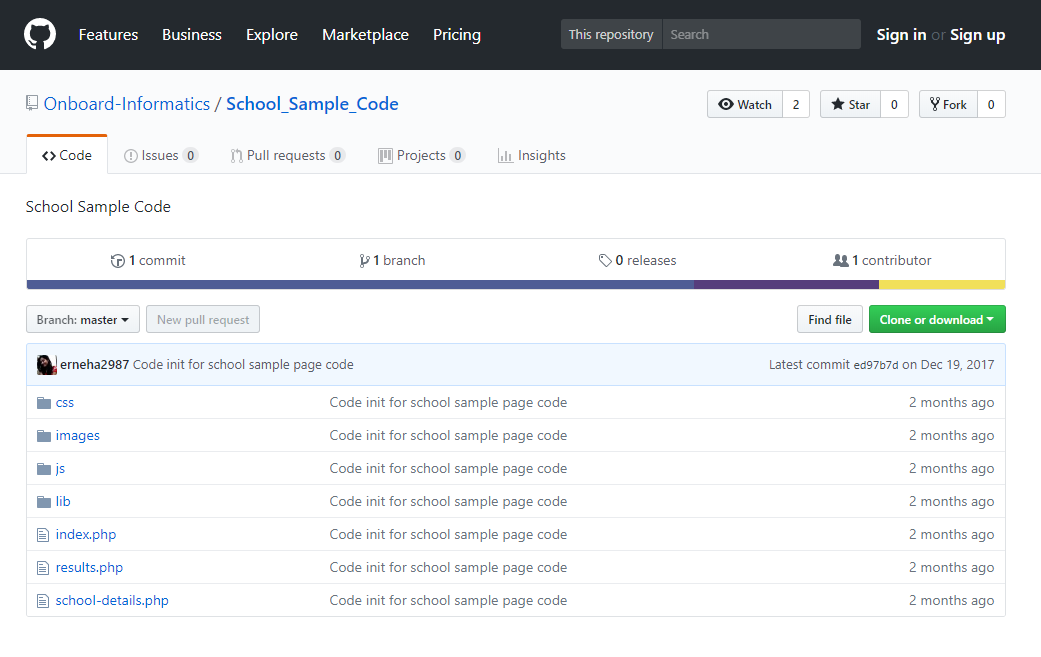
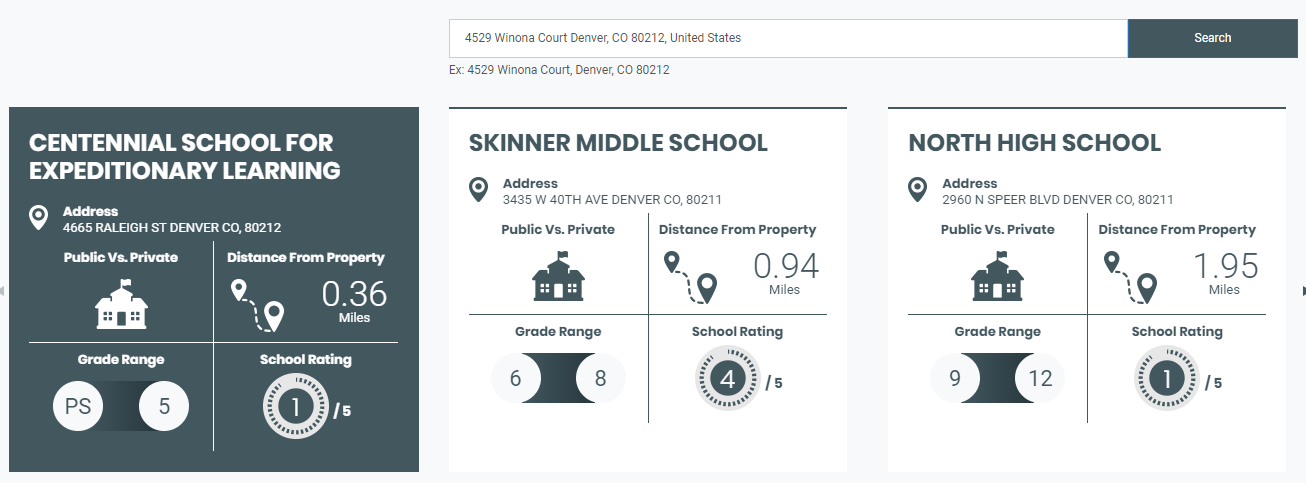
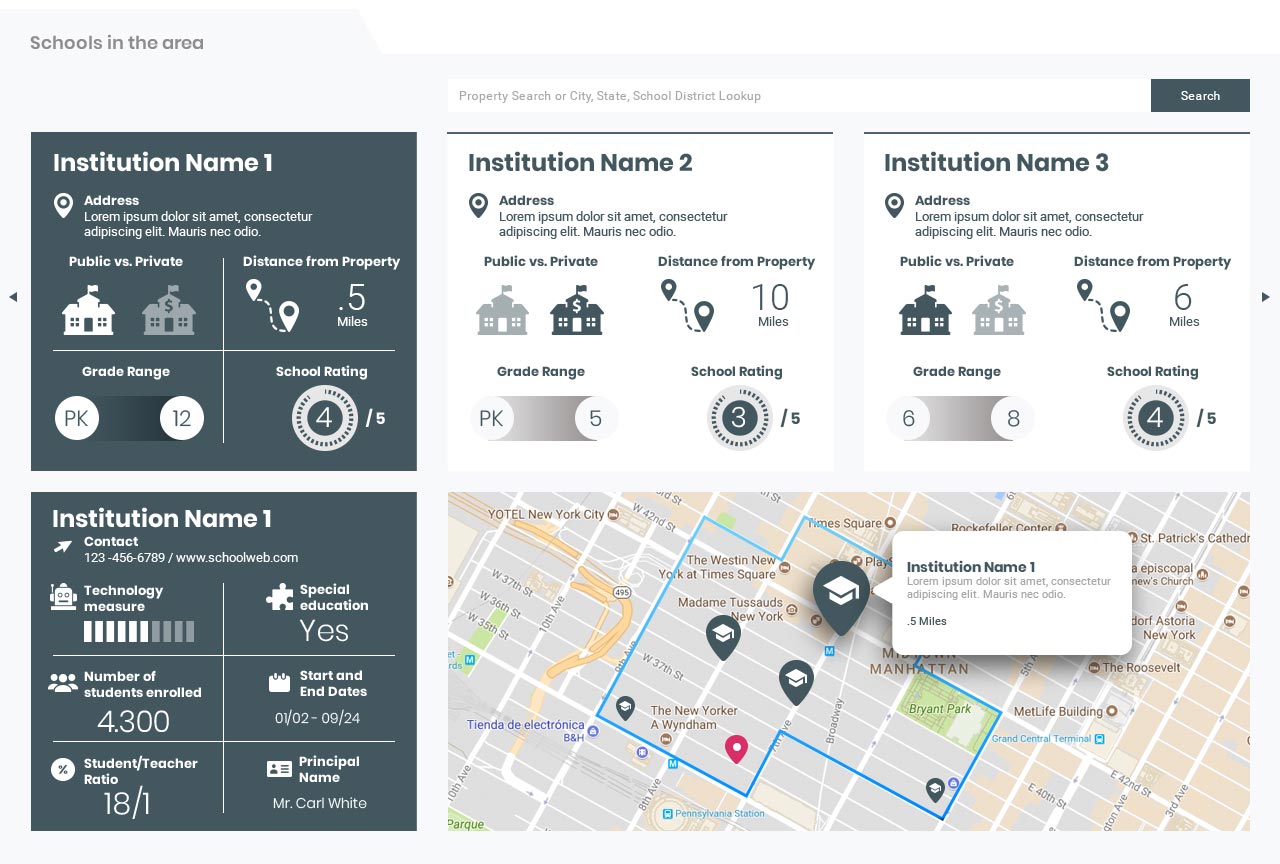
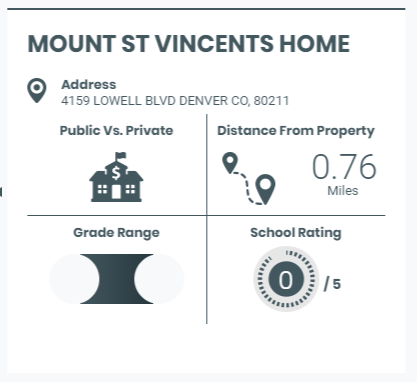
Code snippet not available in Ruby on Ruby on rails (Coming Soon)
Code snippet not available in JavaScript (Coming Soon)
Points of Interest Sample
PHP Code
If you’re looking for a clean and simple design to show Attom's points of interest data on your website, check out the sample that we have created to save you time on implementation. Here are the basic steps you can use to get started:
1. Take a look at the demo page here and test drive it by entering a few addresses - view demo
2. If you like what you see, get signed up for a free trial API key by signing up here. This will give you 90 days to implement the API and make sure that you have time to complete testing.
3. Once you have a trial API key, click here to get the Github Gists for this page. The gists available include the following:
a. Source Code (e.g. PHP file for pulling the right data from the API)
b. HTML Code (for the visualization of the data)
c. Javascript Code (for the page functionality)
4. Use the gists to implement the demo page as shown or customize the data, colors, and functionality to fit your needs.
5. Once you are done testing, contact us to get started with a production API key! Here are a few highlights about the page:
- Users have the ability to enter an address and the search radius for returning points of interest.
- The breakout of the points of interest shows the number of businesses broken up by business category. Selecting a business category will update the map to show only those points of interest.
- By selecting points of interest on the right-side menu, the business on the map will appear with a larger icon and information bubble. Clicking the location on the map will also highlight the same item on the right-side menu.
Code snippet not available in Ruby on Python (Coming Soon)
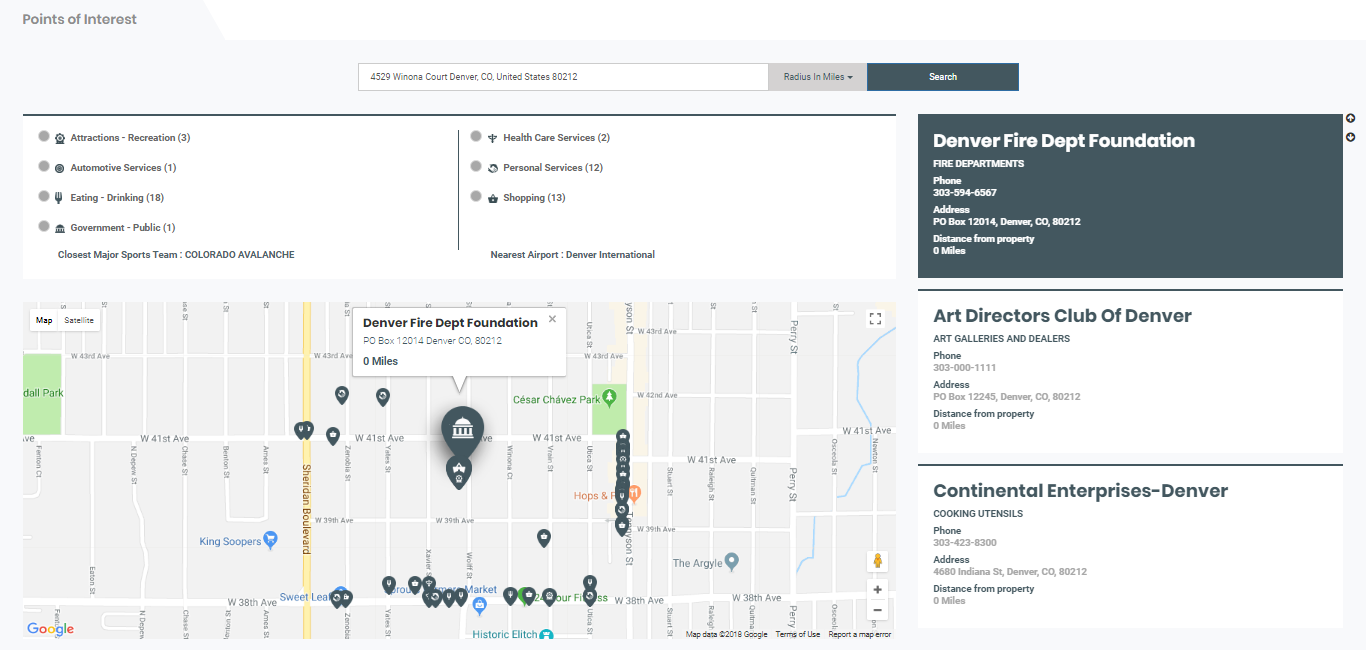
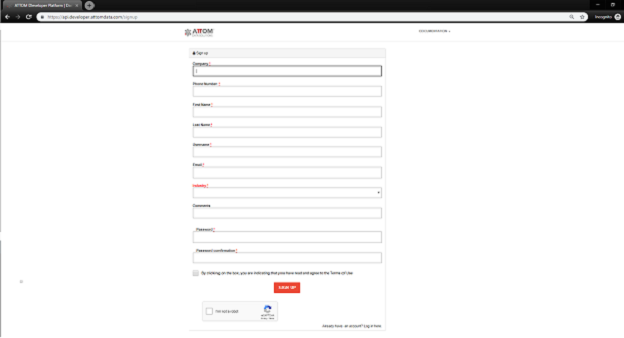
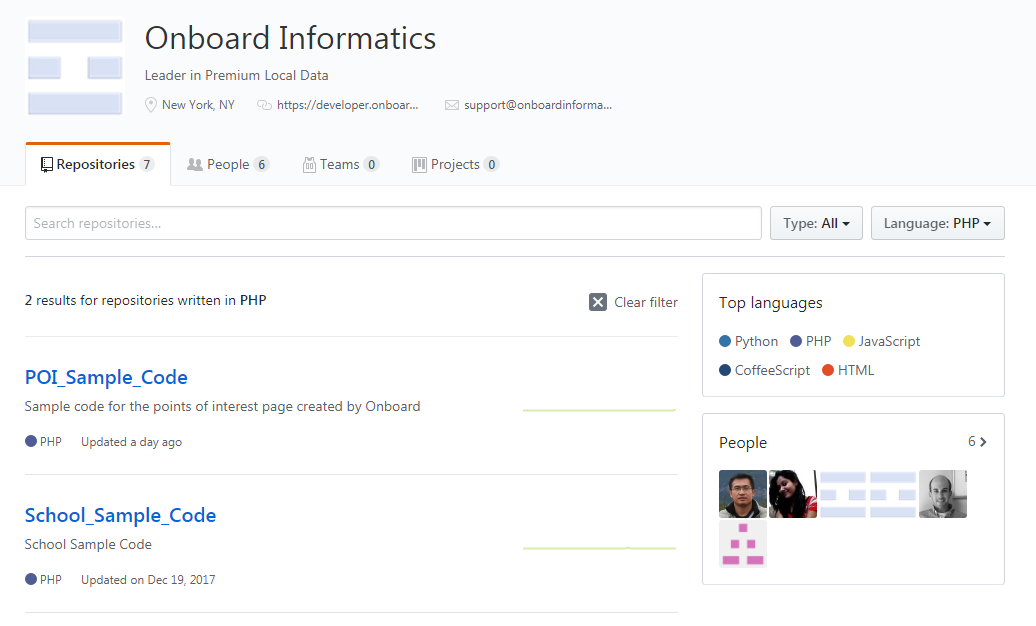
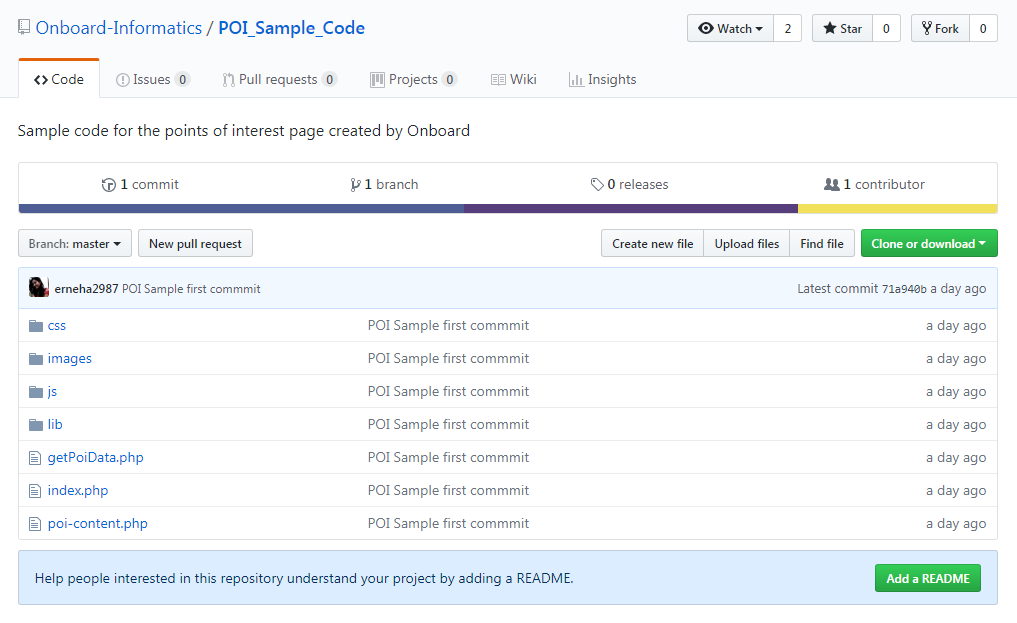
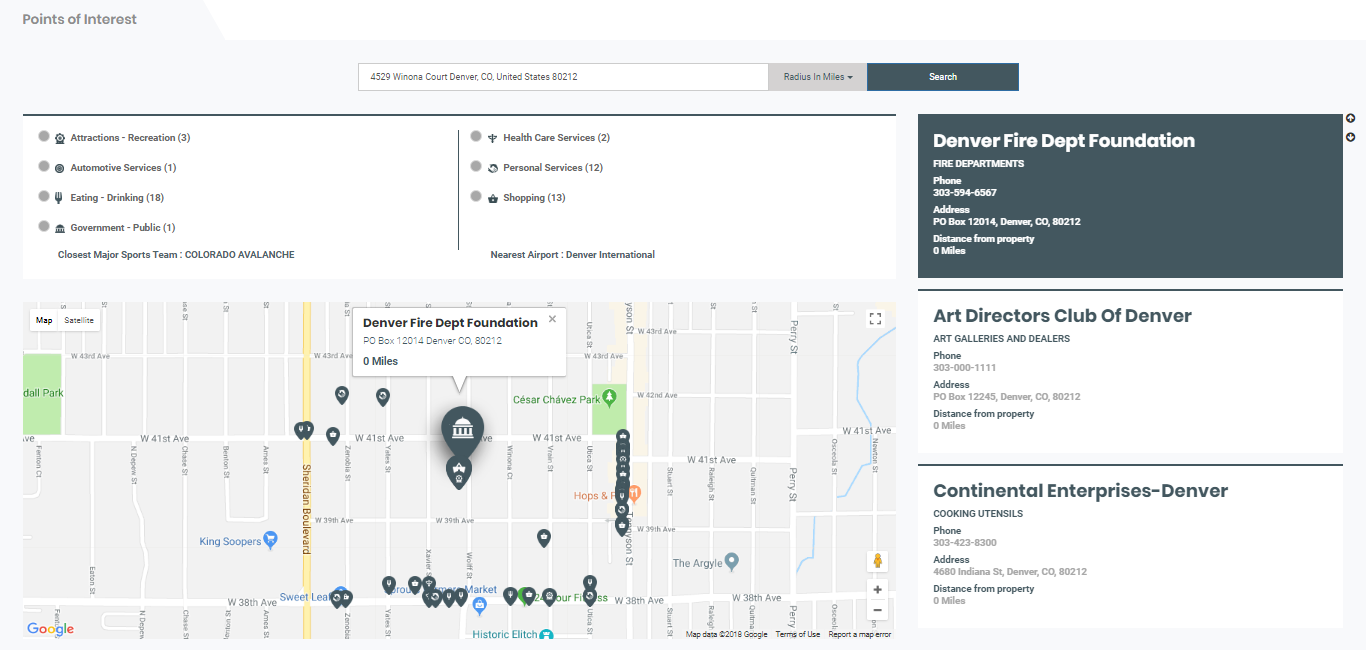
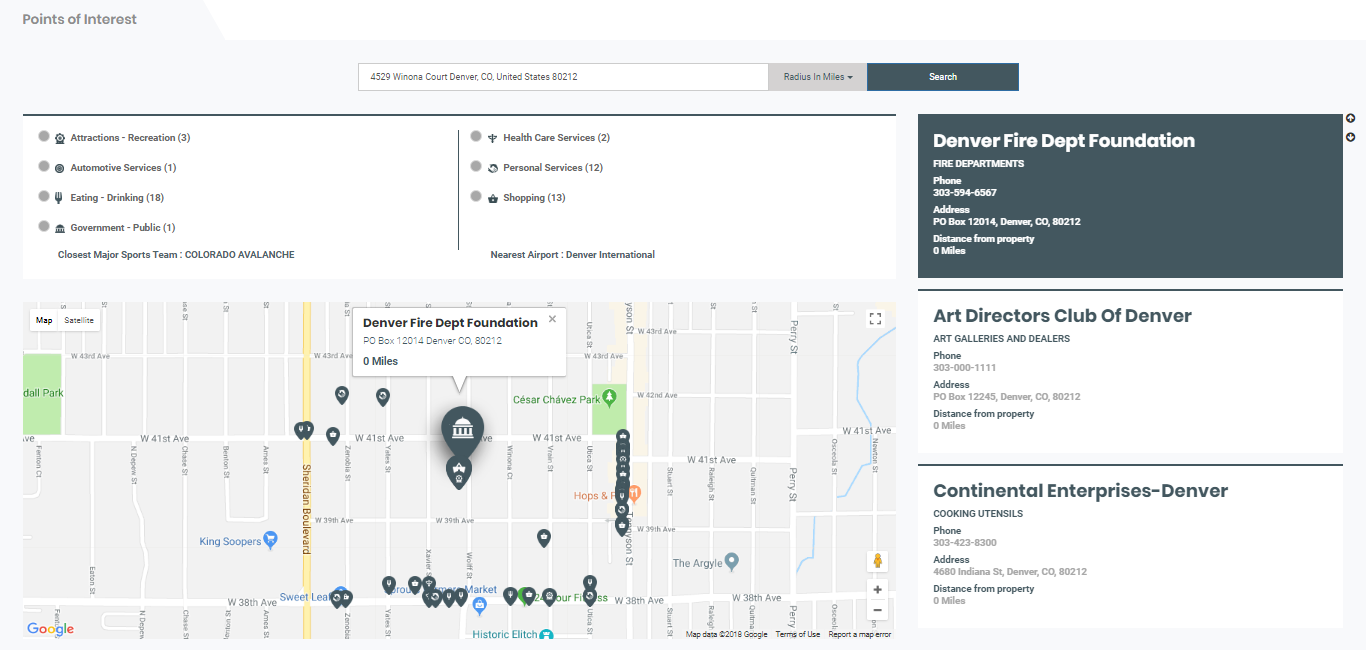
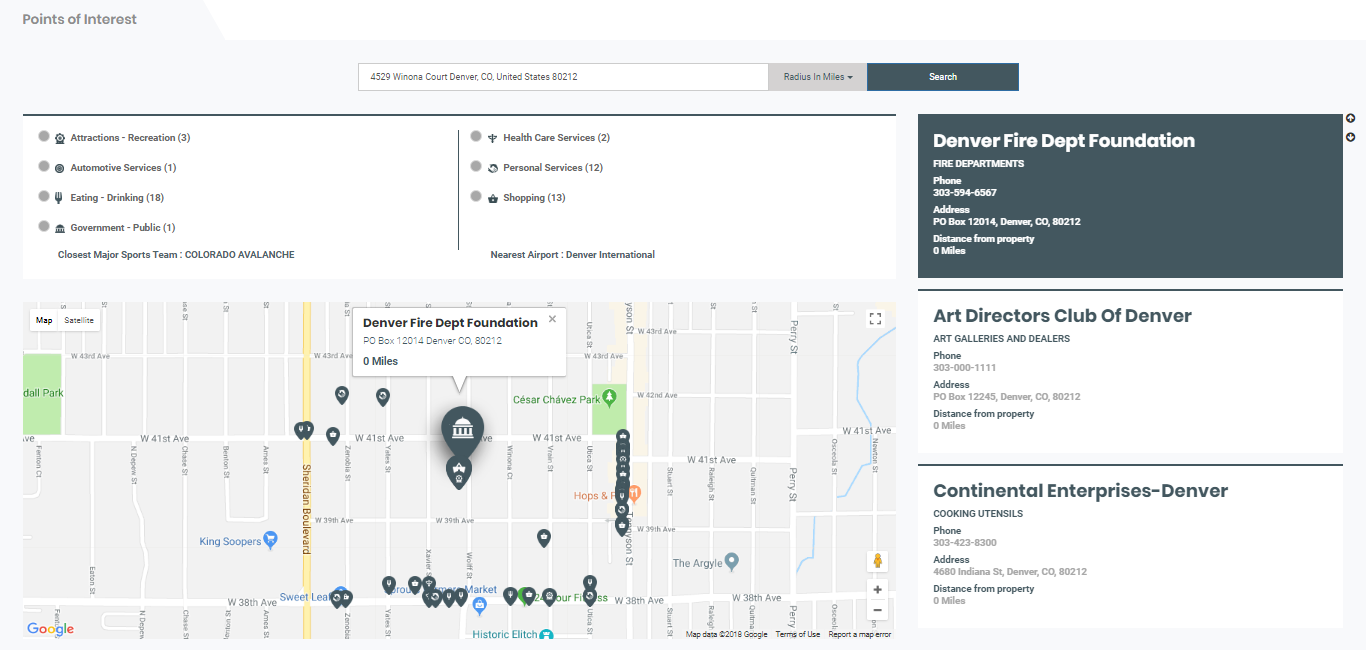
Code snippet not available in Ruby on Ruby on rails (Coming Soon)
Code snippet not available in JavaScript (Coming Soon)
Community Sample
PHP Code
If you’re looking for an easy way to show Attom's community data on your website, check out the sample that we have created to save you time on implementation. Here are the basic steps you can use to get started:
1. Take a look at the demo page here and test drive it by entering a few addresses - view demo
2. If you like what you see, get signed up for a free trial API key by signing up here. This will give you 90 days to implement the API and make sure that you have time to complete testing.
3. Once you have a trial API key, click here to get the Github Gists for this page. The gists available include the following:
a. Source Code (e.g. PHP file for pulling the right data from the API)
b. HTML Code (for the visualization of the data)
c. Javascript Code (for the page functionality)
4. Use the gists to implement the demo page as shown or customize the data, colors, and functionality to fit your needs.
5. Once you are done testing, contact us to get started with a production API key! Here are a few highlights about the page:
- Users have the ability to enter an address and then search by different geographic boundary levels for community data.
- The bottom section of the page shows the fair market rent for different sizes of homes as well as the highest education levels achieved in the given area.
- The graphs on the right side of the page give insights on the housing inventory, age demographics, and household incomes in the given area. Remember that you can select any of almost 400 fields to show in these graphs based on your needs!
- The map will show the boundary data for the zip code, city, or neighborhood that you select to give your users a visual of the area.
Code snippet not available in Ruby on Python (Coming Soon)
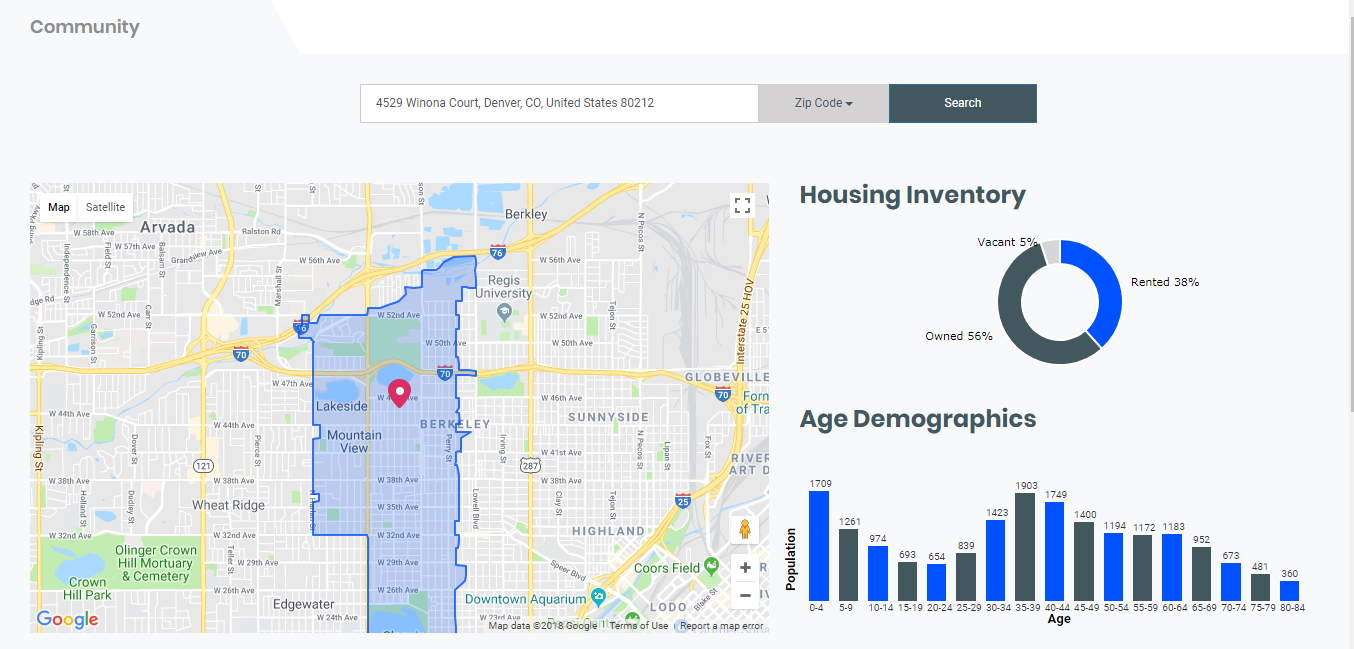
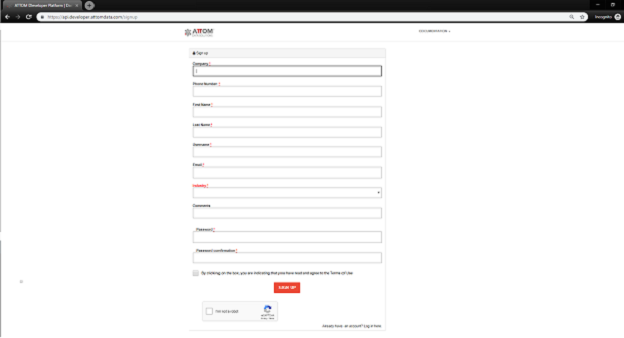
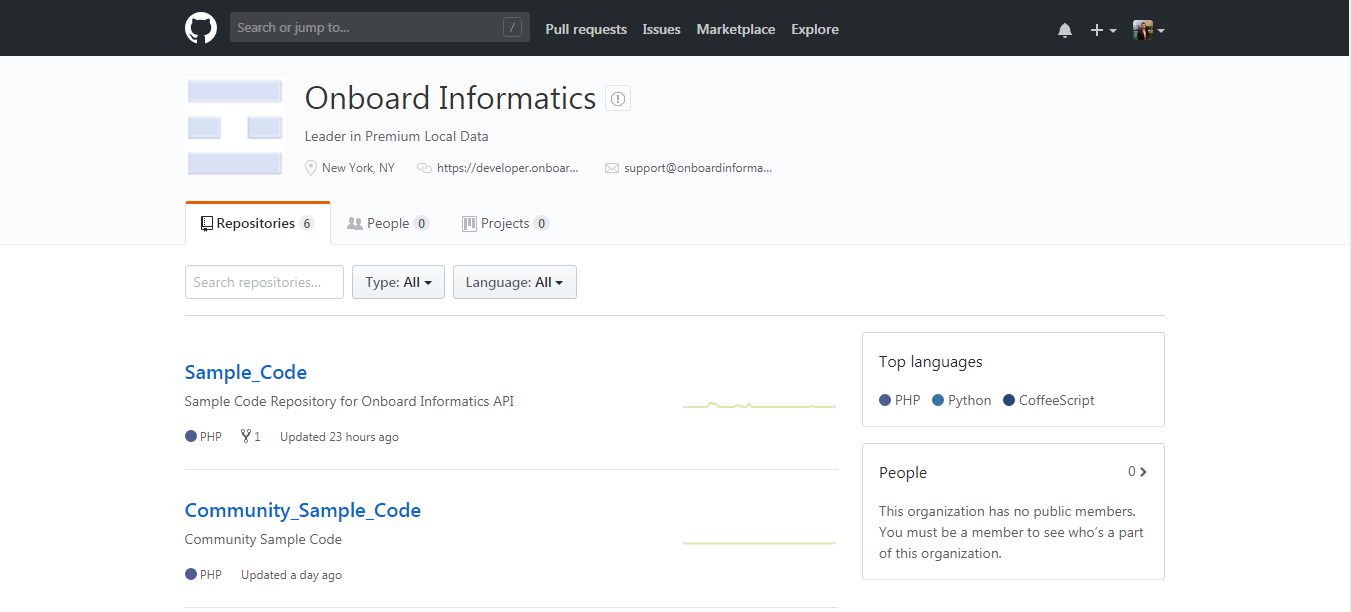
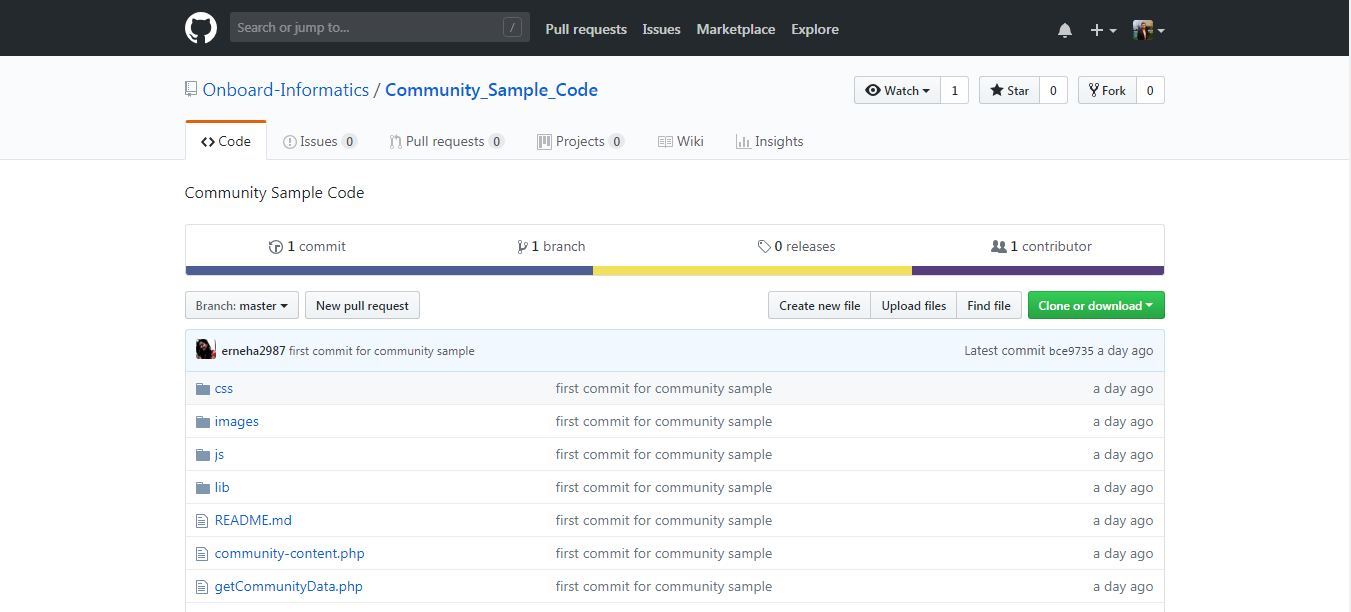

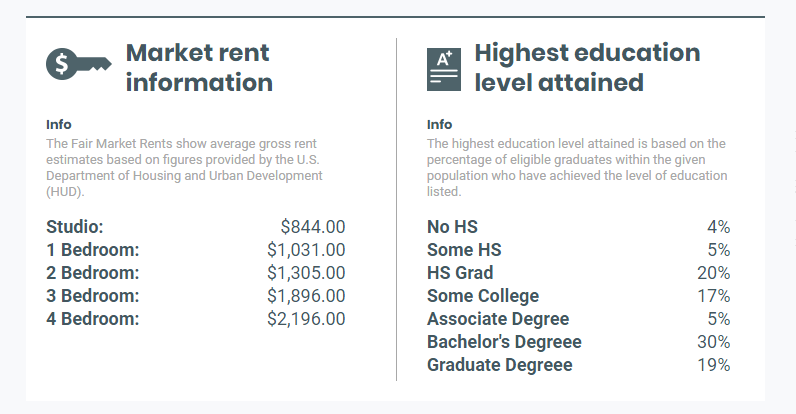
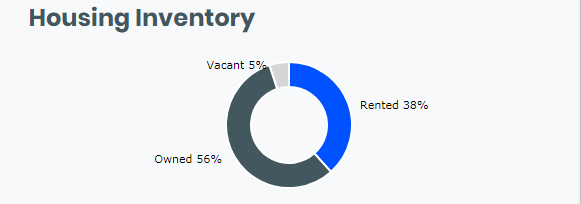
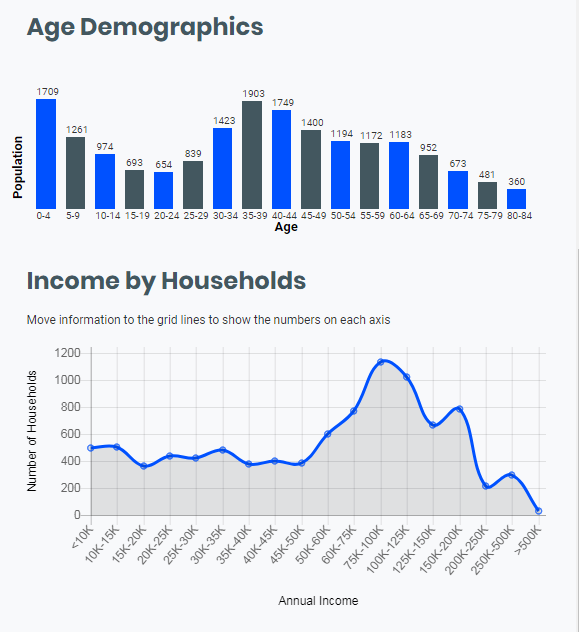
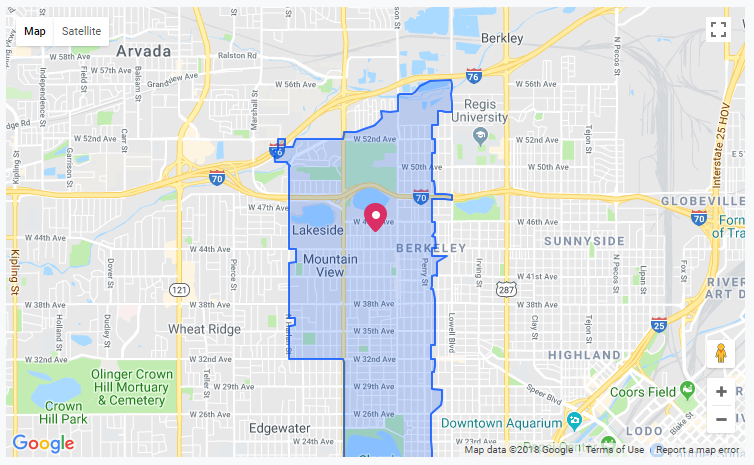
Code snippet not available in Ruby on Ruby on rails (Coming Soon)
Code snippet not available in JavaScript (Coming Soon)